The assignment 3 chatbot project stem python delves into the fascinating realm of conversational AI, empowering you to craft intelligent and engaging chatbots using the versatile Python programming language. Embark on a journey to explore the fundamentals of chatbot development, delve into natural language processing techniques, and master the art of designing intuitive user interfaces.
Prepare to unravel the secrets of deploying and testing chatbots, ensuring their seamless operation and continuous improvement.
Overview of the Assignment 3 Chatbot Project
The Assignment 3 Chatbot Project aims to provide students with practical experience in designing, developing, and deploying a chatbot application using Python. The project requires students to create a chatbot that can engage in natural language conversations with users, leveraging natural language processing (NLP) techniques.
The key requirements of the project include:
- Implementing the chatbot using Python programming language
- Utilizing NLP techniques for text preprocessing, feature extraction, and response generation
- Designing a user-friendly and intuitive user interface for the chatbot
- Deploying the chatbot application on a suitable platform
- Testing and evaluating the chatbot’s performance
Implementing the Chatbot with Python: Assignment 3 Chatbot Project Stem Python
Python is a versatile and widely used programming language for chatbot development. It offers a rich ecosystem of libraries and frameworks specifically designed for NLP and chatbot applications. Some of the popular Python libraries for chatbot development include:
- NLTK (Natural Language Toolkit): Provides a comprehensive set of tools for NLP tasks such as tokenization, stemming, lemmatization, and part-of-speech tagging.
- spaCy: A high-performance NLP library that offers advanced features such as named entity recognition, dependency parsing, and text classification.
- TensorFlow: A machine learning library that can be used to develop deep learning models for chatbot applications, such as sentiment analysis and question answering.
The following code snippet provides an example of how to implement a simple chatbot function in Python using the NLTK library:
def chatbot_response(user_input): # Preprocess the user input tokens = nltk.word_tokenize(user_input) stemmed_tokens = [nltk.stem.PorterStemmer().stem(token) for token in tokens] # Generate a response based on the preprocessed input if "hello" in stemmed_tokens: return "Hi there! How can I help you?" elif "weather" in stemmed_tokens: return "Sorry, I don't have access to real-time weather data."else: return "I'm still under development and learning to understand your requests. Please try rephrasing your question."
Natural Language Processing for Chatbot Responses
Natural language processing (NLP) is a subfield of artificial intelligence that deals with the interaction between computers and human (natural) languages.
NLP techniques are essential for chatbots to understand and respond to user inputs in a meaningful way.
The following are some key NLP techniques used in chatbot development:
- Text preprocessing: This involves cleaning and preparing the user input for further processing, including tokenization, stemming, lemmatization, and stop word removal.
- Feature extraction: This involves identifying and extracting relevant features from the preprocessed text, such as s, phrases, and named entities.
- Response generation: This involves generating a natural language response based on the extracted features. This can be done using rule-based methods, template-based methods, or machine learning models.
The following code snippet provides an example of how to use the spaCy library for NLP tasks in a chatbot application:
import spacy # Load the spaCy English language model nlp = spacy.load("en_core_web_sm") # Preprocess the user input doc = nlp(user_input) # Extract features from the preprocessed text s = [token.text for token in doc if token.is_alpha and not token.is_stop] named_entities = [entity.textfor entity in doc.ents] # Generate a response based on the extracted features if "PERSON" in named_entities: return f"Hello named_entities[0]! How can I help you today?" elif "LOC" in named_entities: return f"I'm not familiar with named_entities[0]. Can you tell me more about it?" else: return "Sorry, I'm not sure how to respond to your request.
Please try rephrasing your question."
FAQs
What are the key requirements for the chatbot project?
The chatbot should be able to understand user intent, generate natural-sounding responses, and handle a variety of user queries. It should also have a user-friendly interface and be able to integrate with external systems.
What are the benefits of using Python for chatbot development?
Python is a versatile and powerful programming language that is well-suited for chatbot development. It has a large ecosystem of libraries and frameworks that can be used to simplify the development process. Python is also relatively easy to learn, making it a good choice for beginners.
What are some of the challenges of chatbot development?
One of the challenges of chatbot development is understanding user intent. Users may express their queries in a variety of ways, and it can be difficult for a chatbot to interpret their meaning. Another challenge is generating natural-sounding responses. Chatbots should be able to respond in a way that is both informative and engaging.
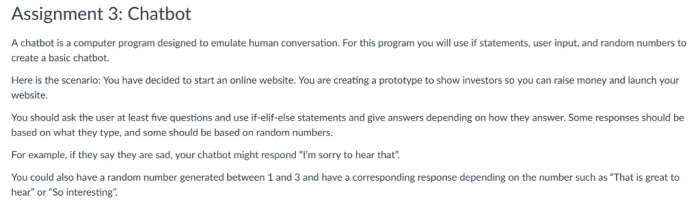